Understanding JSON Web Tokens
As a developer working on modern web applications, you've likely encountered situations where secure and efficient token-based authentication is crucial. JSON Web Tokens (JWT) have become a fundamental component in these scenarios, enabling stateless authentication and seamless communication between services. JWTs provide a compact, self-contained way to transmit information securely between two parties, ensuring data integrity and authenticity without the complexity of managing server-side session states. This guide will walk you through everything you need to know about JWT authentication, from key concepts to best practices, helping you implement it effectively in your applications.
What is JWT?
A JSON Web Token (JWT) is a compact, self-contained way to transmit information between two parties as a JSON object securely. It’s designed to ensure data integrity and authenticity without the need for server-side session management. It is deemed credible because the token's data is digitally signed using a shared secret (HMAC) or public/private key pair (RSA or ECDSA).
JWTs are essential in modern authentication mechanisms and authorization, particularly in stateless environments. Encapsulating user information, such as an email address or an aud claim, within the token eliminates the need for repeated database queries, thereby reducing server load and improving response times. Their small size allows them to be easily transmitted through URLs, POST parameters, or HTTP headers, making them ideal for securing API calls, implementing Single Sign-On (SSO), and managing sessions across distributed systems.
In identity management, JWTs serve as the standard format for ID tokens in protocols like OpenID Connect (OIDC), ensuring efficient and reliable authentication across various platforms.
The Need for Stateless JWTs
As your applications grow in complexity and scale, managing session state on the server can become increasingly challenging. Stateless JWTs offer a solution by embedding all necessary user information within a compact token that accompanies each request. This approach eliminates the need for server-side session storage, allowing for independent verification of each request. By shifting session management to the client, JWTs reduce server load, enhance scalability, and improve the efficiency and responsiveness of your authentication process—particularly in distributed systems.
Structure of a JSON Web Token
Every JWT consists of three key components: the Header, the Payload, and the Signature. These parts are Base64Url encoded and combined to form the final token.
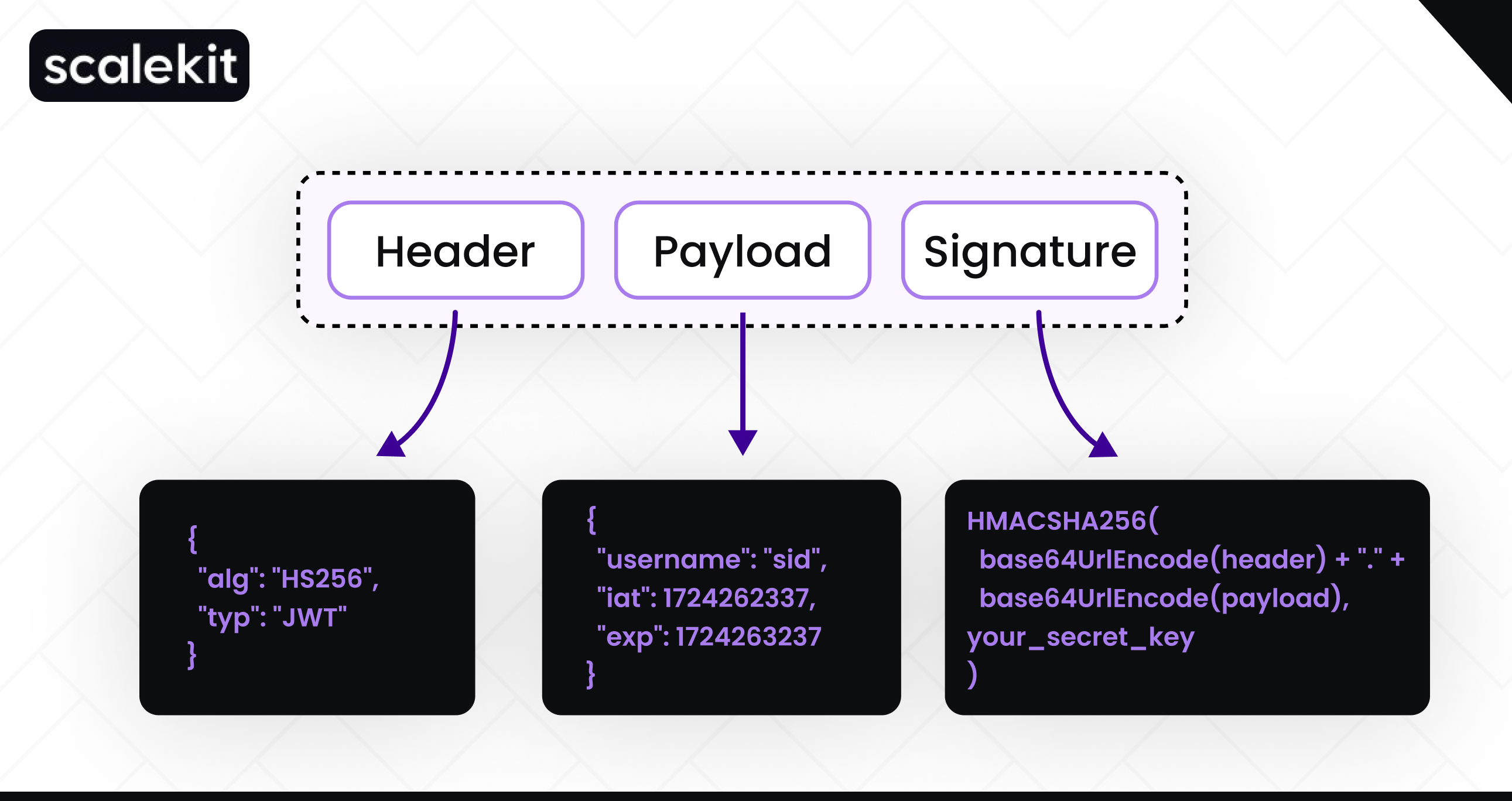
- Header: The header typically includes the token type (JWT) and the signing algorithm (e.g., HMAC SHA256 or RSA). It sets the foundation for how the token will be verified.
- Payload: The payload contains the claims—statements about the user or additional data you must include. These claims can be registered, public, or private. It’s crucial to choose the correct claims and manage sensitive data carefully.
- Signature: The signature ensures the token's integrity. It's generated by combining the encoded header, payload, a secret key, and the specified algorithm. This prevents tampering and verifies the token’s authenticity.
Key Factors for Secure JWT Implementation
While the structure is fundamental, understanding how JWTs handle claims, validation, and signing keys is equally essential. The Header, Payload, and Signature work together to create a secure, verifiable token, but how you manage these elements—like choosing the correct signing algorithm or validating the token against your keys—will determine the effectiveness of your JWT implementation.
Example of a JWT
Here’s an example of what a JWT looks like:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VybmFtZSI6InNpZCIsImlhdCI6MTcyNDM5Njc1NSwiZXhwIjoxNzI0Mzk3NjU1fQ.Be-R35pRrGVMADGmxNHYbGGkZVSVUdbwQGtPTNxC0-Y
- Header: eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9
- Payload: eyJ1c2VybmFtZSI6InNpZCIsImlhdCI6MTcyNDM5Njc1NSwiZXhwIjoxNzI0Mzk3NjU1fQ
- Signature: Be-R35pRrGVMADGmxNHYbGGkZVSVUdbwQGtPTNxC0-Y
How JWT Authentication Works
When a user logs into your application, the server generates a JWT and sends it to the client, typically stored in local storage or a cookie. For subsequent requests, this token is included in the Authorization header. The server validates the token’s signature and, if it’s valid, processes the request while maintaining a stateless authentication system. This process simplifies authentication across distributed systems by eliminating the need for server-side session management, thus enhancing the efficiency and scalability of your authentication flow.
While storing JWTs in local storage is common, it poses security risks like token hijacking. For better security, it's recommended that JWTs be stored in HTTPOnly and Secure cookies. The best practices section below provides more details on secure storage practices.

Validate JSON Web Tokens
When your server receives a JWT, the token must be validated to ensure its authenticity. This involves checking the signature against the token’s header and payload using the specified algorithm. Additionally, claims like expiration (exp) and issuer (iss) should be validated to confirm that the token hasn’t expired and is coming from a trusted source.
Signing Keys
The security of your JWTs depends on the signing keys used to create their signatures:
- Symmetric Keys (HMAC): With HMAC, the same key is used for both signing and verifying the token. This means that both the issuer and the verifier must share this secret key. It's crucial to keep this key secure because if it is compromised, anyone with access to the key can forge valid tokens.
- Asymmetric Keys (RSA): Asymmetric cryptography uses a pair of keys: a private key and a public key. The private key is used to sign the token, and this key must be kept secret. The corresponding public key is then distributed to anyone who needs to verify the JWT's authenticity. This method offers enhanced security, especially in distributed systems, as only the private key holder can create valid tokens, while anyone with the public key can verify them.
Safeguarding your signing keys is critical to maintaining the integrity of your JWTs. Without proper key management, the security of your entire authentication system could be compromised.
JSON Web Key Sets (JWKS)
If your application involves rotating keys or managing multiple signing keys, JSON Web Key Sets (JWKS) can streamline this process. JWKS is a JSON-based data structure that represents a set of cryptographic keys. This allows you to efficiently manage and distribute various keys, automating the process and enhancing security without manual intervention.
For example, a JWKS might look like this:
{
"keys": [
{
"kty": "RSA",
"kid": "1e9gdk7",
"use": "sig",
"n": "oahUI...wKJH",
"e": "AQAB"
},
{
"kty": "EC",
"kid": "2h9gdk8",
"use": "sig",
"crv": "P-256",
"x": "f83OJ3D2...aMn3rnJL",
"y": "x_FEzRu9...JHDFHJ3G"
}
]
}
In this example, the JWKS contains two keys: one RSA key and one elliptic curve key. Each key is identified by a kid (key ID) and contains the necessary parameters for verifying JWTs. Your application's JWKS endpoint can provide these keys to clients or services that need to verify your JWTs, allowing them to fetch the latest keys as they rotate.
Access Tokens
In many cases, JWTs serve as access tokens, controlling who can access specific resources. These tokens include claims that specify what actions the token holder is authorized to perform. By validating these claims, your server ensures that only users with the correct permissions can access sensitive data or perform specific actions.
Implementing JWT: A Practical Guide with Node.js
Let's set up a basic Node.js project to create and manage JWTs. This example will focus on the backend API, so we'll skip the frontend view engine setup (like EJS) and focus on RESTful API implementation. If needed, a React.js frontend can be integrated later.
1. Initialize the Node.js Project
First, we need to set up a basic Node.js project. We’ll install the necessary packages for routing, JWTs, password hashing, and cookies. Here’s how you get started:
npm init -y
npm install express jsonwebtoken bcryptjs body-parser cookie-parser ejs
These packages are our toolkit for this project. Express will handle our routes, jsonwebtoken will help us create and verify JWTs, bcryptjs will handle password hashing, body-parser will parse incoming request bodies, cookie-parser will manage cookies, and ejs will render views.

2. User Login

Let’s assume the user already exists in the system. When a user logs in, we validate their credentials, and if everything checks out, we generate JWTs and manage their session.
Here’s how we handle user login:
app.post('/login', async (req, res) => {
const { username, password } = req.body;
const user = users.find(u => u.username === username);
if (!user) {
return res.status(401).json({ message: 'Invalid username or password' });
}
const isPasswordValid = await bcrypt.compare(password, user.password);
if (!isPasswordValid) {
return res.status(401).json({ message: 'Invalid username or password' });
}
const accessToken = jwt.sign({ username }, SECRET_KEY, { expiresIn: '15m' });
const refreshToken = jwt.sign({ username }, SECRET_KEY, { expiresIn: '7d' });
// Store tokens in secure, HTTP-only cookies
res.cookie('token', accessToken, { httpOnly: true, secure: true });
res.cookie('refreshToken', refreshToken, { httpOnly: true, secure: true });
return res.json({ message: 'Login successful' });
});
During login:
- The user's credentials are checked against the stored data.
- If they match, new JWTs are generated.
- These tokens are stored securely in HTTP-only cookies to ensure JavaScript can't access them and are only sent over HTTPS.
- The server then responds with a JSON message confirming the login.
3. Protecting Routes
Now, let’s ensure that only authenticated users can access certain parts of our app. We’ll use middleware to check the JWT on protected routes.
const authenticateToken = (req, res, next) => {
const token = req.cookies.token;
if (!token) {
return res.status(401).json({ message: 'Access denied. No token provided.' });
}
jwt.verify(token, SECRET_KEY, (err, user) => {
if (err) {
return res.status(403).json({ message: 'Invalid token' });
}
req.user = user;
next();
});
};
app.get('/dashboard', authenticateToken, (req, res) => {
res.json({ message: `Welcome, ${req.user.username}!` });
});
What’s Happening:
- The authenticate Token middleware checks if there’s a token in the cookies.
The user can proceed to the protected route (like /dashboard) if the token is valid.
4. Token Refresh and Logout
To keep the user’s session going without making them log in again every 15 minutes, we use a refresh token.
Token Refresh:
app.post('/token', (req, res) => {
const refreshToken = req.cookies.refreshToken;
if (!refreshToken) {
return res.status(401).json({ message: 'No refresh token provided' });
}
jwt.verify(refreshToken, SECRET_KEY, (err, user) => {
if (err) {
return res.status(403).json({ message: 'Invalid refresh token' });
}
const accessToken = jwt.sign({ username: user.username }, SECRET_KEY, { expiresIn: '15m' });
res.cookie('token', accessToken, { httpOnly: true, secure: true });
return res.json({ accessToken });
});
});
What’s Happening:
- We verify the refresh token and, if it’s valid, issue a new access token.
- The new access token is stored in a secure, HTTP-only cookie.
Logout:
app.get('/logout', (req, res) => {
res.clearCookie('token');
res.clearCookie('refreshToken');
return res.json({ message: 'Logged out successfully' });
});
What’s Happening:
- When the user logs out, we clear the tokens from the cookies, effectively ending the session.
5. Verifying JWTs with JWT.io
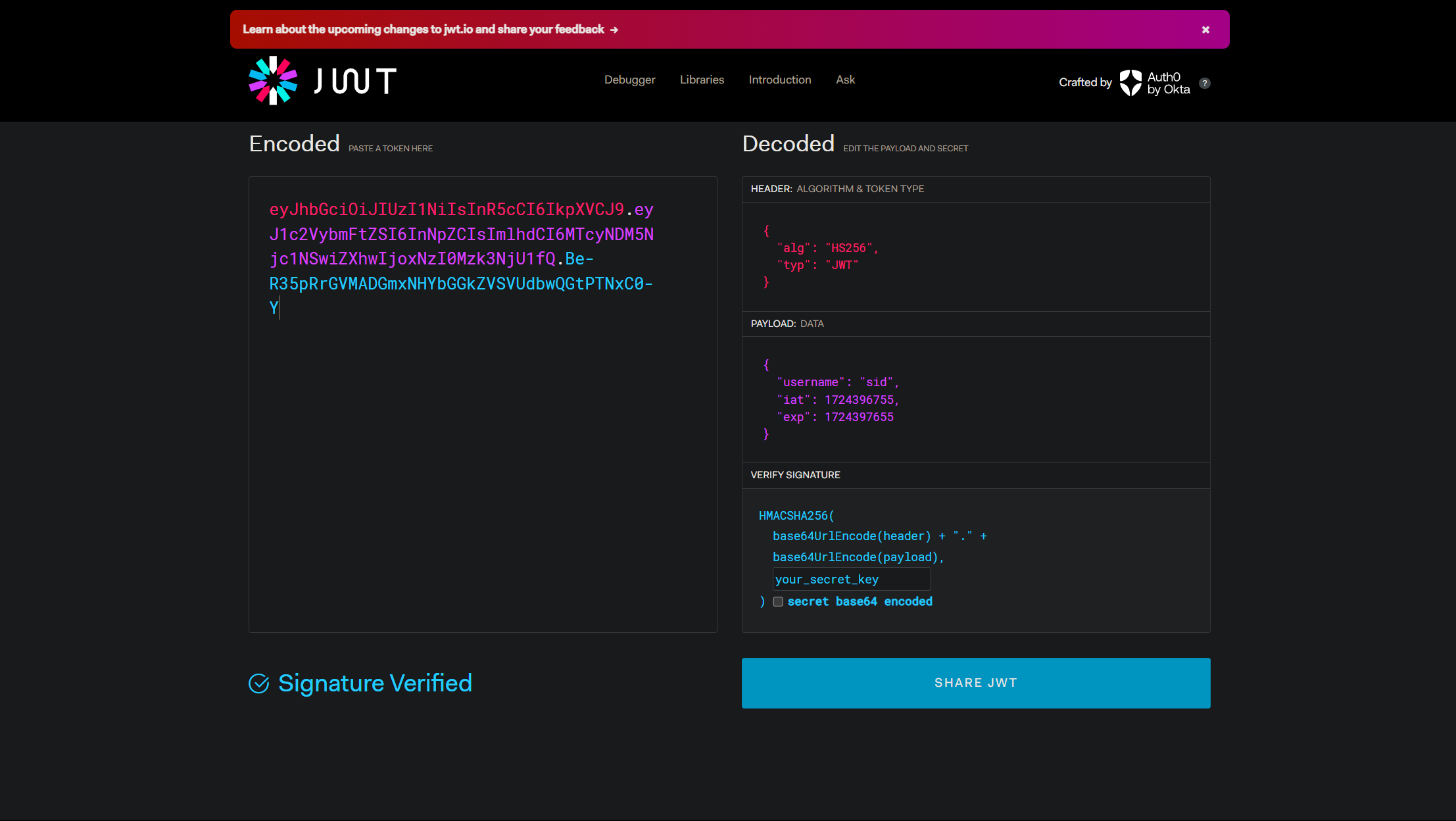
Finally, to ensure your JWTs are working as expected, you can use a tool like JWT.io. Just paste your JWT into the tool, and it’ll decode the payload and verify your signature.
Expanding to Other Languages
While the example above uses Node.js, the principles of JWT implementation remain the same across different languages. Here’s a brief overview of how you can implement similar functionality in other environments:
- Python: Use PyJWT for creating and verifying tokens.
- Java: Leverage libraries like jjwt for JWT handling.
- Go: Use the golang-jwt package to manage tokens.
Each language has its best practices and tools, but the core concepts—creating, signing, and verifying JWTs—remain consistent.
Why Use JWTs? The Key Benefits
JWTs bring a host of benefits that make them a go-to choice for developers:
- Stateless Simplicity: With JWTs, there’s no need to worry about managing session storage on the server. Each token is self-contained, carrying all the information needed for authentication. This simplifies your architecture and makes scaling your application much more manageable.
- Boosted Performance: JWTs are compact by design, which means they can be transmitted quickly over the network. This efficiency translates into faster request handling and a smoother user experience, especially in applications that demand speed.
- Cross-Platform Flexibility: One of the standout features of JWTs is their platform independence. Whether you’re working with Java, Python, Node.js, or something else, JWTs integrate seamlessly, allowing you to maintain consistency across different environments.
- Built-In Security: Every JWT is signed, ensuring its data hasn’t been tampered with. This signature verification gives you confidence that the token is legitimate and that your users’ information remains protected. You can use JSON Web Encryption (JWE) to encrypt the token's payload for additional security. By combining JWT with JWE, you ensure the token's integrity and authenticity and safeguard sensitive data within the token, making it inaccessible even if intercepted.
Case Study: Lessons from Real-World JWT Security Breaches
Understanding JWT’s security risks is essential, as real-world incidents have shown. For instance, a critical flaw in the jsonwebtoken library in early 2023 allowed remote code execution due to improper JWT verification. Another vulnerability involved the misuse of the "none" algorithm, which could bypass signature verification entirely. These cases highlight the importance of keeping JWT libraries up-to-date and ensuring secure algorithm configurations. By learning from these breaches, you can avoid similar pitfalls in your implementations.
Best Practices for Using JWTs
To get the most out of JWTs while keeping your application secure, it's crucial to follow these best practices:
- Secure Storage: Always store JWTs securely on the client side. The best approach is to use HTTP-only cookies to prevent JavaScript from accessing the tokens and help reduce the risk of cross-site scripting (XSS) attacks. Additionally, ensure that these cookies are marked as secure, meaning they are only transmitted over HTTPS. This prevents the data encoded in the JWT from being intercepted during transmission. Implementing these simple measures can significantly enhance the protection of your tokens from theft.
- Token Expiration: Setting an expiration time (exp claim) on your JWTs is essential. Short-lived tokens reduce the window of opportunity for attackers if a token is compromised. Pairing short lifespans with refresh tokens allows you to extend user sessions securely while limiting the potential damage from an exposed token.
- Token Lifecycle Management: JWTs typically go through phases—valid, expired (often up to 24 hours), and cleared (after 24 hours). Rather than focusing on blacklisting tokens, it’s crucial to implement a strategy that respects these phases. After expiration, tokens should be cleared from client storage and treated as invalid by the server. Depending on your implementation, you might use JWT metadata or service-specific mechanisms to handle expired tokens, ensuring they cannot be reused.
- Sensitive Data Storage: Never store sensitive information in the JWT payload. Remember, JWTs are base64 encoded, not encrypted, meaning that anyone who gets hold of the token can easily decode it. Keep sensitive data out of the payload to minimize the risk of exposure.
Keep JWTs Lightweight: Resist the temptation to load JWTs with excessive information, even though they can reduce the need for database queries. Overloading JWTs with data can lead to larger token sizes, increasing latency and impacting performance. Stick to the essentials to maintain efficiency and security.
Scenarios & Use Cases for JWT
- OpenID Connect (OIDC) for Identity Management: JWTs play a critical role in OpenID Connect (OIDC), serving as the format for ID tokens. These tokens are used to verify a user's identity and provide authentication data to client applications. OIDC leverages JWTs to facilitate a secure, decentralized approach to identity management, enabling seamless user authentication across multiple platforms. The self-contained nature of JWTs ensures that the authentication process remains efficient and reliable, even in distributed environments.
- Session Management in Modern Applications: While JWTs are often associated with stateless authentication, they can also be used effectively in session management scenarios. For instance, in hybrid systems that combine stateless and stateful sessions, JWTs can securely maintain session data across different services. This is particularly useful in applications that need to scale while ensuring session continuity, such as e-commerce platforms or enterprise applications requiring consistent user sessions across multiple devices. Additionally, in a microservice-based architecture, where a single request might traverse multiple services during its lifecycle, JWTs play a crucial role. Each service can independently validate the authenticity of a request by verifying the JWT, ensuring secure and consistent authentication across a truly distributed architecture. This approach enhances security and scalability by decentralizing the authentication process, allowing each service to manage its own authentication needs.
- Securing API Endpoints with JWT: JWTs are commonly used to secure API endpoints by embedding authentication and authorization details within the token itself. This approach ensures that each API request carries the necessary credentials, allowing for quick verification without the need for repeated server-side checks. By using JWTs, developers can protect APIs from unauthorized access and streamline the communication between client applications and backend services.
- Single Sign-On (SSO) Across Platforms: JWTs are a natural fit for Single Sign-On (SSO) solutions, allowing users to authenticate once and gain access to multiple services. The ability to carry authentication information across different platforms with a single token simplifies the user experience and reduces the overhead of managing multiple login credentials. JWT-based SSO is particularly beneficial in environments where users must interact with various applications within the same ecosystem, such as corporate intranets or cloud-based service platforms.
Security Risks and When Not to Use JWT
While JWTs offer many benefits, they aren’t without risks. If someone steals a JWT, they can impersonate the user until the token expires. Mitigating this requires secure transmission (HTTPS), proper storage (HTTP-only cookies), and additional safeguards like IP whitelisting. JWT may not be suitable if your application requires instantaneous token revocation or extensive server-side session management. Consider traditional server-side session handling in these cases, which might offer better control over session states.
Conclusion
JSON Web Token (JWT) is a powerful, secure, stateless authentication tool in modern web applications. By following best practices—such as safe storage, setting expiration times, and understanding the risks—you can implement JWT effectively and enhance your application’s security. Start applying these strategies today to ensure robust and reliable system authentication.
FAQ
1. What is JWT used for?
A JSON Web Token (JWT) is a way to securely send information between different parties as a compact JSON object. It’s widely used in web applications for authentication and authorization, allowing for stateless session management, securing API endpoints, and enabling Single Sign-On (SSO) across multiple platforms. JWTs help ensure that the data being exchanged is trustworthy and hasn’t been altered, so they’re a go-to solution in modern web development.
2. Is JWT an authentication method?
Yes, JWT is commonly used as an authentication method in web applications. While it’s not an authentication protocol, JWT is a token format that works within frameworks like OAuth 2.0. By embedding user claims in the token, JWTs help verify a user’s identity and grant access to resources. JWTs can be used in cookies or as Bearer Tokens in HTTP headers to authenticate requests, enabling secure, stateless authentication without the need for the server to keep track of the session state.
3. Is JWT an API?
No, JWT isn’t an API. A JSON Web Token (JWT) is a compact, URL-safe token format designed to transmit information between parties securely. While it’s often used within APIs to handle user authentication and authorize access to resources, JWT is just the token, not an API.
4. When should JWT be used?
You should use JWT when you need a secure, stateless way to transmit information between parties. It’s perfect for stateless authentication in microservices, securing API endpoints, and enabling Single Sign-On (SSO) across various platforms. However, if your application needs instant token revocation, heavy server-side session management, or storing sensitive data securely within the token, JWT might not be the best fit.