Enterprises use Ping Identity to manage secure access to their applications for their employees. In case your SaaS app is on its way to win an enterprise customer, you should support Single Sign-on (SSO) capability.
In this guide, let’s look at how you can add SSO to your SaaS app using React and NodeJS techstack. Towards the end of this guide, you will learn how to authenticate enterprise users using Ping Identity and successfully log into your applications.
Why SSO matters
Imagine your team juggles Outlook, Asana, and Confluence daily, each requiring a separate login. This creates password fatigue, security risks, and a high operational burden for IT teams.
With SSO, users log in once and seamlessly access multiple applications, which helps enhance login security by simplifying user identity verification and digital credential management. This tutorial will guide you through implementing SSO in a Node.js + React app using Ping Identity, with Scalekit simplifying authentication management.
What you'll build
By the end of this guide, you’ll:
- Identify a user’s identity provider and verify end users' identities to authenticate them
- Handle the authentication flow and manage session tokens
- Securely log the user into your app using their Ping Identity credentials
Let’s get started! Follow along with the code using this GitHub repository. The README.md file provides setup instructions for local development.
Ping Identity in your auth flow
Authenticating your enterprise users takes a different approach. In this approach, enterprise users come to your app and click on “continue with SSO”. They should be redirected to Ping Identity to further authenticate, ensuring robust login security, and successfully log into your app.

Our current application has two components—Backend and frontend. For the purposes of this guide, they are powered by NodeJS and ReactJS respectively.
Here are the problems in our current approach:
- Your application needs to establish a connection with Ping Identity over protocols such as SAML or OIDC. These are time-consuming to implement and delays onboarding your enterprise customer.
- Your application is closely tied with Ping Identity, and may create overhead to further support more IdPs such as Microsoft Entra ID or Okta in case your future customers use them.
- There is too much friction between your application, Ping Identity, and enterprise users. It’s far too risky to compromise on security.
There’s a better way.
Simplify auth flow with Scalekit
Scalekit abstracts these problems by providing a user-friendly solution. Your application redirects enterprise users to Scalekit, and waits for a callback to receive user profile details on successful verified logins.
Here’s how it looks:

All the API interactions are scoped between your app and Scalekit. It reduces many security risks, and it’s way easier to ship SSO. Perhaps in hours!
Add Scalekit to your project
To interact and authenticate with Scalekit, let’s use the Scalekit SDK. Use the following terminal command to install Scalekit SDK in your backend.
Install Scalekit SDK:
The example app has a backend with package.json that lists Scalekit as its dependency. Hence, running npm run install-all would install dependencies for both frontend and backend as listed in their instructions.
API credentials as environment variables
The SDK requires API credentials associated with your account. Sign up for an account and grab the details from the API Config section.
Rename .env.example to .env with following environment variables:
Set up environment variables.
Shell/terminal
This step sets your application to seamlessly interact with Scalekit.
Overview of steps to implement SSO
Scalekit orchestrates the SSO flows with Ping Identity. To use Scalekit in your application, here’s what the end-to-end flow looks like.
- The React UI should direct the enterprise user to an authorization URL with necessary identifiers.
- Scalekit automatically identifies the enterprise user’s IdP to Ping Identity and presents an authentication method mandated by their organization.
- On successful authentication, your application will receive the user profile details that Ping Identity will send you, allowing you to store digital credentials securely.
Time to implement authorization URL and handle successful callbacks.
Configuring Scalekit for SSO
Step-by-step setup in Scalekit
Before integrating SSO into your application, Scalekit must be configured correctly. Follow these steps:
1. Create an Organization in Scalekit
- Log in to your Scalekit Dashboard.
- Navigate to Organizations and Create Organization.
- Copy the Organization ID for later use.
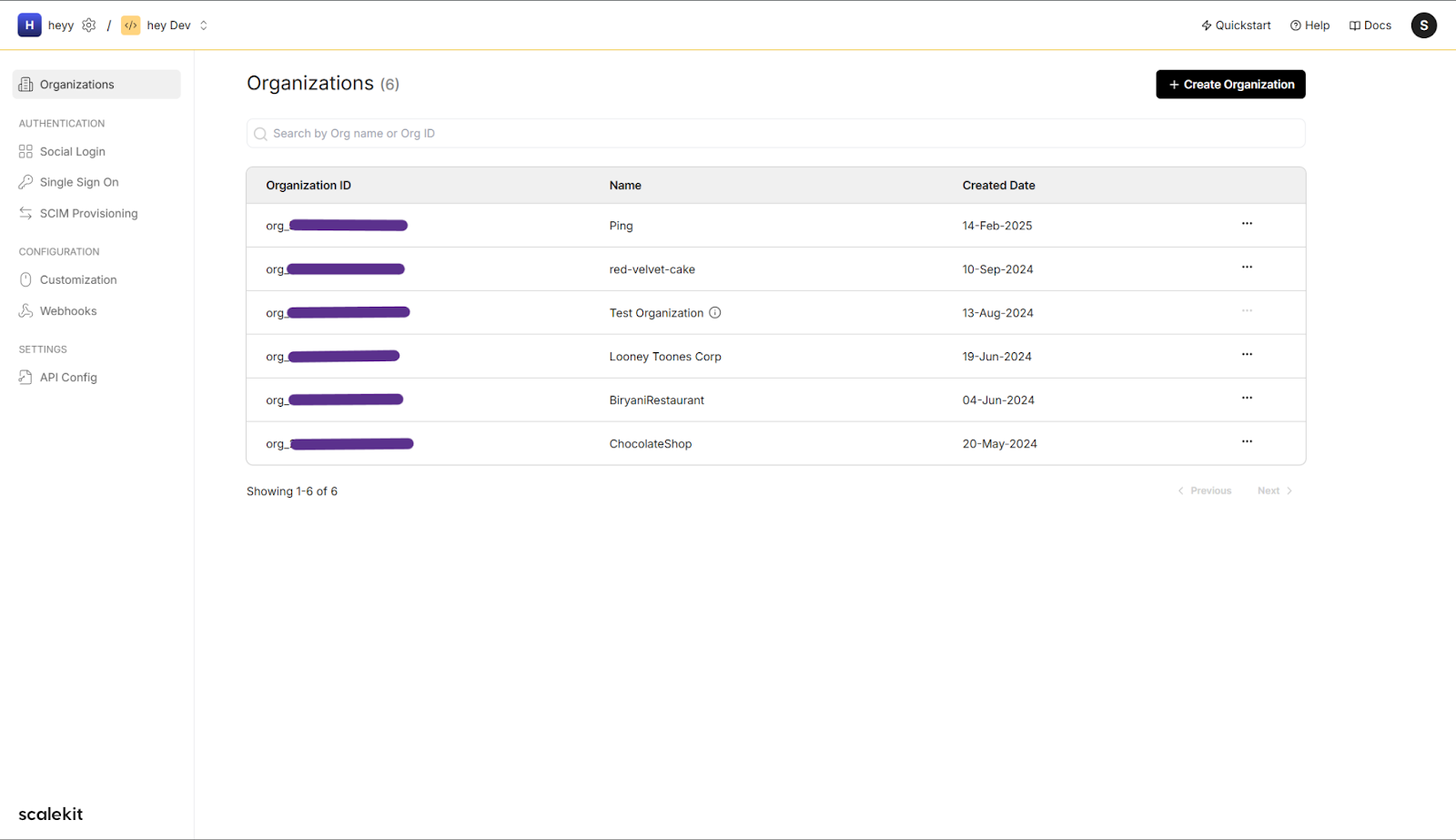
2. Register Ping Identity as an Identity Provider
- Navigate to your new Organization on the dashboard.
- Click on Single Sign On and select Ping Identity as the IdP and SAML as the protocol. This will help in securely managing users’ digital identities.
- Follow the steps shown and configure the IdP.
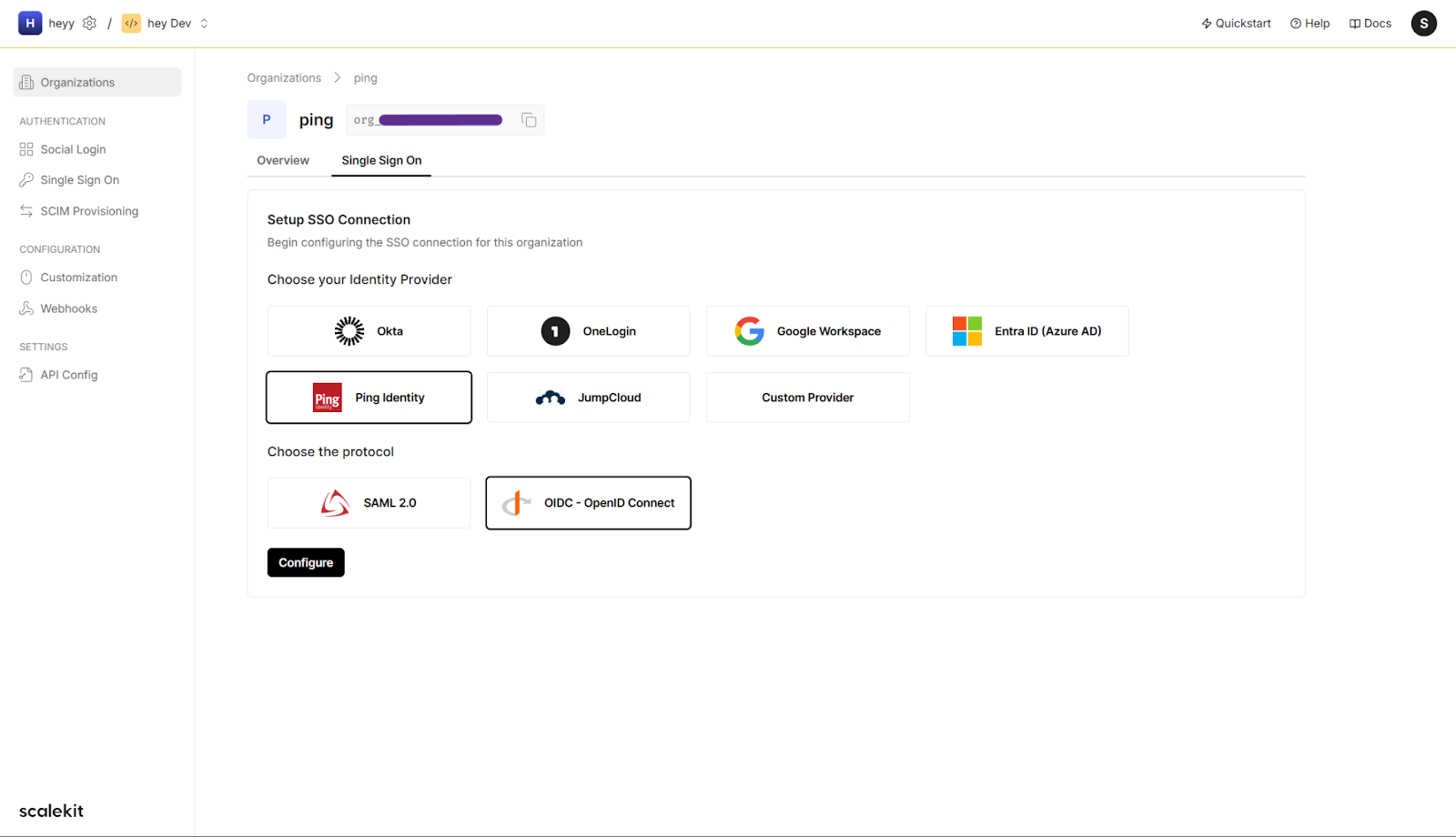
3. Whitelist redirect URI
- Navigate to API Config in Scalekit.
- Add your app’s redirect URI (e.g. http://localhost:3000/callback for local development).
- Save changes.
4. Retrieve API credentials
- In API Config, copy the Client ID and Client Secret.
- Add these credentials to your file.
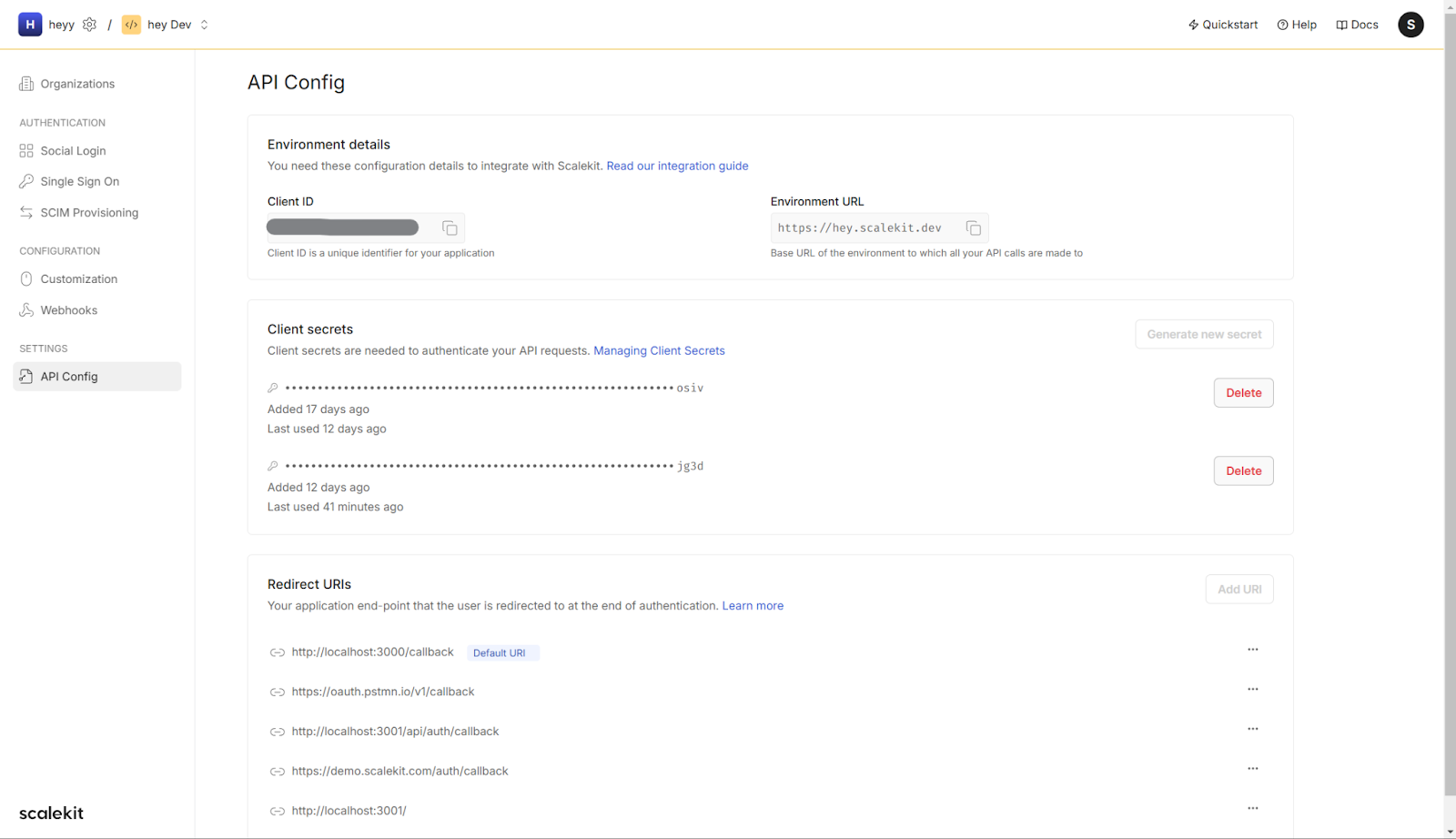
Auth destination to handle user profile details
In the backend/routes/index.ts, the /login route generates an authorization URL using Scalekit sdk and instructs the browser to follow it to Identity Provider—in this case, Ping Identity.
To create a destination URL, you need to pass the redirect URI and a couple of options to Scalekit’s getAuthorizationUrl() method.
Any ONE of connectionId, organizationId or loginHint must be passed in order to initiate single sign-on. These are available once you create an organization from Scalekit Dashboard. These resemble your customers. For ease of testing for developers, you can use a default “Test Organization”.
Note that the redirect URI you will pass should be registered in the Scalekit Dashboard > API Config.
For demonstration purposes, the example app takes these details as part of environment variables. In real-time, these identifiers will be used at the runtime based on your app’s business use case.
While connectionId and organizationId are the identifiers that Scalekit gives to your app at the runtime, a user can give his/her email address, for instance john@example.org. We will later see React frontend sending these details to the backend.
💡 Pro tip: If you use any @example.com or @example.org domains for testing purposes, Scalekit initiates SSO with an IdP Simulator. This simulates Ping Identity.

Once successfully authenticated, your application gets a callback from Scalekit with a “code”. Your application needs to exchange it for the user profile details.
(This is an excerpt from) backend/routes/index.ts
You can use these details to store it securely. This example uses browser session storage which may not be ideal for all production scenarios.
Initiate SSO at the React login
Now that we have /login endpoint that redirects the enterprise users to authenticate, it’s time for our application’s UI to initiate single sign on.
Go to frontend/src/components/Login.jsx
Make a call to the /login endpoint and redirect the user by changing window.location.href at runtime.
Once SSO is complete, the example app displays the user profile details. See frontend/src/pages/Dashboard.jsx on how it renders this information.
Debugging SSO authentication Issues
Even after a successful setup, authentication failures can occur due to misconfigurations or permission issues. Below are some common SSO errors, their causes, and how to fix them.
Onboard the enterprise
Your application is all set up to handle SSO authentication.
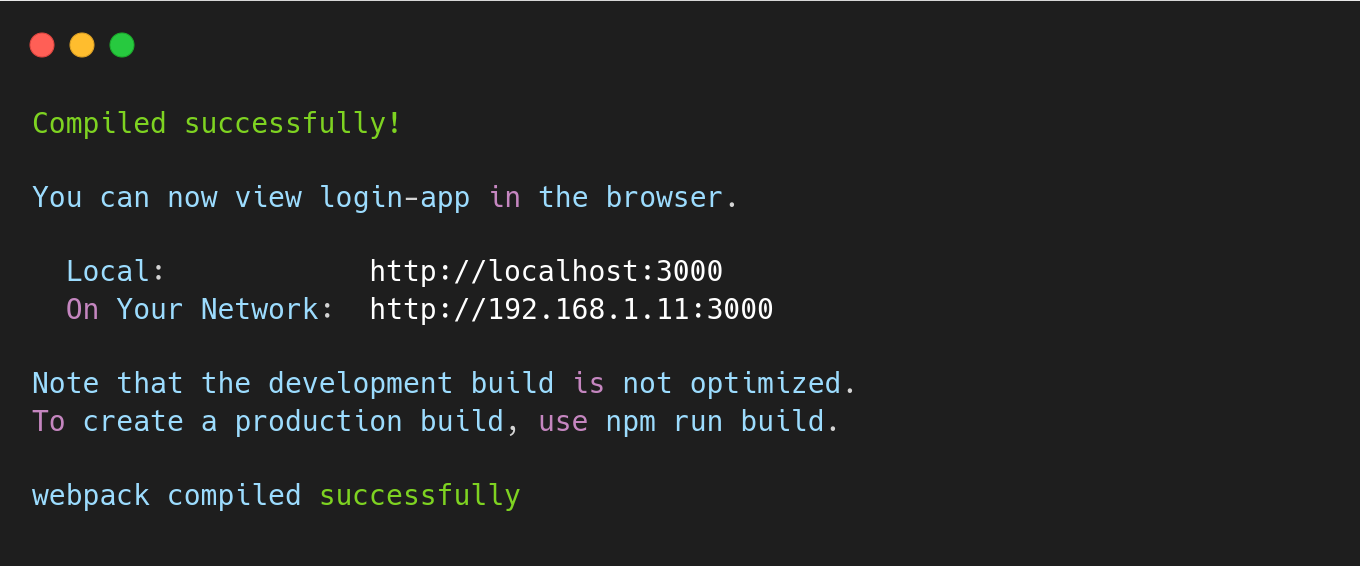
Now, it’s time to connect Scalekit with Ping Identity for your enterprise customer. This step is typically handled by an IT administrator, who configures the connection by entering specific details into Ping Identity.
The administrator will:
- Retrieve configuration details such as the SAML Metadata URL, Client ID, and Redirect URI from the Scalekit Dashboard.
- Enter these details into Ping Identity, allowing it to recognize and authenticate users for your application.
- Define user access policies within Ping Identity to control which users can log in through SSO.
With these steps completed, enterprise users will be able to authenticate through Ping Identity and access your application securely.
Successful implementation
You have now implemented SSO with Ping Identity in a React and Node.js application using Scalekit. Your application successfully identifies enterprise users, handles authentication, and securely logs them in—all in just a few seconds.
Scalekit provides dedicated environments for both development and production, so you can test your integration safely before rolling it out. Once everything is working as expected, you can switch to production from Workspace > Environment in the top navigation menu.
💡 Pro tip: Notice idp_initiated_login in the example code? Read this guide to allow enterprise users to log in to your app directly from the Ping Identity applications list.
FAQ
What is the difference between SSO and PingFederate?
The Single Sign-On technology allows users to establish a single authentication during which they can access connected applications by bypassing repeated authentication processes. PingFederate operates as a Ping Identity business product that handles SSO protocol execution for SAML as well as OIDC protocols.
What is the difference between identity and SSO?
A combination of username and password functions as identifying credentials in user account systems. Users accessing different applications avoid repeated logins when they authenticate just once through SSO.
Are SSO and OIDC the same?
No, they are different. Users receive advantages through SSO because the process provides authentication for multiple apps with a single login. The OIDC (OpenID Connect) protocol aids secure authentication by providing protocols to facilitate contemporary SSO application deployment.
Can I use Scalekit for both SSO and Social Logins?
Yes, Scalekit supports both enterprise SSO (Ping Identity, Okta, Microsoft Entra ID) and social logins (Google, GitHub, Facebook, etc.).
Can I test SSO without an enterprise IdP?
Yes, Scalekit offers an IdP Simulator that allows developers to test SSO implementations without the need to configure multiple Identity Providers during development.